Hibernate
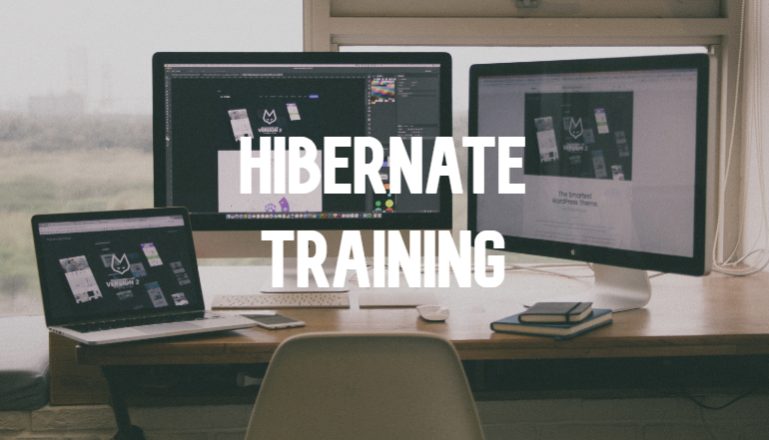
1. What is Hibernate?
Hibernate ORM (or simply Hibernate) is an object-relational mapping tool for the Java programming language. It provides a framework for mapping an object-oriented domain model to a relational database. Hibernate handles object-relational impedance mismatch problems by replacing direct, persistent database accesses with high-level object handling functions.
Hibernate is free software that is distributed under the GNU Lesser General Public License 2.1.
Hibernates primary feature is mapping from Java classes to database tables, and mapping from Java data types to SQL data types. Hibernate also provides data query and retrieval facilities. It generates SQL calls and relieves the developer from the manual handling and object conversion of the result set.
2. Why Hibernate?
Minimize Code Changes
Hibernate minimizes code changes when we add a new column to a database table.
JDBC
- You have to add a new field in your POJO class.
- Change your JDBC method that performs the “select” to include the new column.
- Change your JDBC method that performs the “insert” to add a new value into the new column.
- Change your JDBC method that performs the “update” to update an existing value in your new column.
Hibernate
- Add the new field into your POJO class.
- Modify the Hibernate XML mapping file to include the new column.
Thus, database table changes can be easily implemented using Hibernate with less effort.
Reduce Repeat Code
Hibernate reduces the amount of repeating lines of code, which you can often find with JDBC. For your understanding, I have left a simple scenario below.
Lazy Loading
We can achieve lazy loading using Hibernate. Consider an example where there is a list of users in the user table. The identity proof documents uploaded by the users are stored in the identity proof table. The user has a ‘one to many’ relationships with the identity proof. In this case, the user is the parent class and identity proof is the child class. If you fetch the parent class, i.e the user, all the documents associated with the user will also be fetched. Imagine the size of each document. As the number of documents increases, the size of data to be processed also increases, and hence it will slow up the application.
Avoiding Try-Catch Blocks
JDBC will throw SQLexception, which is a checked exception. So you will be writing “try-catch” blocks in your code. Hibernate handles this by converting all JDBC exceptions to unchecked exceptions. Therefore, you need not waste your time implementing try-catch blocks.
Versioning
Database versioning is an important feature of Hibernate. Hibernate enables developers to define version type fields in an application, which is updated when data is updated every time. The advantage is if two different users retrieve the same data and then modify it, and one user saved their modified data to the database before the other user, the version is updated. Now when the other user tries to saves their data, it will not allow it because this data is not up to date. In JDBC, this check is performed by the developer.
Audit Functionality
Hibernate provides a library called “Envers” which enables us to easily gain audit functionality. It creates tables and stores the audited data with version number from where we can track the changes made to the entity. Let me explain how auditing is done using Envers.
JPA Annotation Support
Hibernate provides support to JPA annotations like @Entity, @Table, @Column, etc. These annotations make the code portable to other ORM frameworks. You can find some of the annotations explained with an example in this link.
Connection Pooling
As explained on Wikipedia, connection pooling is a mechanism in which the database connections, when created are stored in the cache by an external tool so that these connections can be reused from the cache when the application tries to connect to the same database in future. Connection pooling helps increase performance. We can achieve this connection pooling in Hibernate. Hibernate supports the following pools:
3. How to prepare?
There are two distinct actions if you’re looking to complete a transaction in hibernate:
• Commit
• Rollback
Once a transaction is committed, the transaction data is written to the database. In case there is a rollback, the data exchange is flushed and never written or updated to the database.
Hibernate consists of three different types of relationships:
• One-to-One mapping
• One-to-Many mapping
• Many-to-Many mapping
Hibernate keeps a log of every data exchange with the help of a transaction. Thereon, in case a new exchange of date is about to get initiated, the function Session.beginTransaction is executed in order to begin the transaction.
An XML document is where object/relational mappings are usually defined in. This mapping file gives instructions to hibernate on how to accurately map the defined class/classes to the database tables. In addition, the format .hbm.xml. Should be used to save the file with the mapping document.
Lazy loading is defined as a technique in which objects are loaded on an on-demand basis. It has been enabled by default since the advent of Hibernate 3 to ensure that child objects are not loaded when the parent is.
HQL allows you to express joins in four ways:
• An implicit association join
• A fetch join in the FROM clause
• A theta-style join in the WHERE clause
• An ordinary join in the FROM clause
Automatic dirty checking can be defined as a feature that helps us in saving the effort of explicitly asking Hibernate to update the database every time we modify or make changes to the state of an object inside a transaction.
Hibernate has numerous ways of managing concurrency. They are as listed below:
• Automatic versioning
• Detached object
• Extended user sessions
There are five distinct collection types that are used in hibernate for one-to-many relationship mappings.
• Bag
• Set
• List
• Array
• Map
4. Important materials:
a. E-books:
Hibernate Recipes: a Problem-Solution Approach by Gary Mak, Srinivas Guruzu
High-Performance Java Persistence by Vlad Mihalcea
Java Persistence With Hibernate
b. Videos:
Hibernate full course:
Hibernate introduction:
Hibernate tutorials for beginners:
5. Sample test papers:
http://www.allindiaexams.in/engineering/cse/hibernate-mcq-quiz-hibernate-online-test
6. Certifications on Hibernate:
1. Hibernate Courses & Training Online (Udemy)
This e-learning platform has compiled a list of over 25 courses that will help you to enhance your skills in this framework irrespective of your current proficiency levels. The classes are divided based on difficulty level namely beginner, intermediate and advanced. The bestsellers on the platform are Spring & Hibernate for beginners, Java persistence, fundamentals, spring data JPA using Hibernate, create a crud application and more. Check out our compilation of Best Spring, Boot & MVC Tutorials.
2. Hibernate Programs & Classes (Pluralsight)
Pluralsight has a combination of beginner and intermediate courses to help you to bridge the gap between an object-oriented domain model and a traditional relational database. The classes provide an introduction to the configuration, mapping and querying and the opportunity to get hands on.
3. Persistence with Hibernate Certification Training (Edureka)
This self-paced course by Edureka is designed to provide you an understanding of the fundamental concepts of Hibernate. Work on topics like sessions, transactions, associations, mappings, inheritance as well as advanced features such as NoSql, Spring, Filter, Search and Validator. Following the theoretical lectures solve complex RDBMS problems and enhance your skills to become an expert in this area.
4. Hibernate Courses & Training (LinkedIn Learning – Lynda)
This platform helps you to stay ahead in the field with these expert-led courses. You may choose to complete both of the training ones by one or just choose the one that fits your experience level. Create solutions for Java environments and provide persistent data that can be stored and retrieved at a later time apart from working on the fundamental concepts.
6. Important tips on Hibernate:
1. Find performance issues with Hibernate Statistics
Finding the performance issues as early as possible is always the most important part. The main issue with that is, that most of the performance issues are hardly visible on a small test system. They are caused by some small inefficiencies which are not visible, if you test with a small database and only one parallel user on your local test system.
2. Improve slow queries
Slow queries are not a real JPA or Hibernate issue. This kind of performance problems occurs with every framework, even with plain SQL over JDBC and needs to be analyzed and fixed on the SQL and database level. If you do this, you will quit often recognize that you can not do these more complex or optimized SQL queries with JPQL or the Criteria API.
3. Choose the right Fetch Type
Another common issue is the usage of the wrong Fetch Type. It is specified in the entity mapping and defines when a relationship will be loaded. Using the wrong Fetch Type can result in a huge number of queries that are performed to load the required entities.
4. Use query specific fetching
As I explained in Tip 3, you are not able to define the optimal Fetch Type for all queries in the entity mapping. The good thing is, that you don’t need to do that
5. Use caches to avoid reading the same data multiple times
Modular application design and parallel user sessions often result in reading the same data multiple times. It is quite obvious that this an overhead that you should try to avoid. One way to do this is to cache the data that it often read and not changed to often.